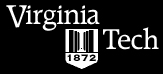
You will use the concept of inheritance for this project.
You will read in some data from an input file and then create student objects based on that data. The data contained in these objects should then be printed out using the toString method of the Student class. The toString method should be modified to allow printing each student as indicated below. Sample input and output is shown below.
Requirement: You are required to have three classes in this project with the following names: Student, Person and Test. The Test class should contain the code for reading the data in from the input file and creating one Student object per input line. It is also where the processed data should be printed out.
Buggs Bunny: 123 Disney World, Orlando, FL 32801: (321)123-4567: buggsbunny@disney.com: Freshman: Donald Duck: 124 Disney World, Orlando, FL 32801: (321)123-4568: donaldduck@disney.com: Freshman: Mickey Mouse: 125 Disney World, Orlando, FL 32801: (321)123-4569: mickeymouse@disney.com: Senior: Roadrunner: 126 Disney World, Orlando, FL 32801: (321)123-4570: roadrunner@disney.com: Junior: Minnie Mouse: 127 Disney World, Orlando, FL 32801: (321)123-4571: minniemouse@disney.com: Sophomore:All output should be written to a file called output.txt. A sample output file is shown below:
================================================= Name: Buggs Bunny Address: 123 Disney World, Orlando, FL 32801 Phone Number: (321)123-4567 Email: buggsbunny@disney.com Status: Freshman ================================================= ================================================= Name: Donald Duck Address: 124 Disney World, Orlando, FL 32801 Phone Number: (321)123-4568 Email: donaldduck@disney.com Status: Freshman ================================================= ================================================= Name: Mickey Mouse Address: 125 Disney World, Orlando, FL 32801 Phone Number: (321)123-4569 Email: mickeymouse@disney.com Status: Senior ================================================= ================================================= Name: Roadrunner Address: 126 Disney World, Orlando, FL 32801 Phone Number: (321)123-4570 Email: roadrunner@disney.com Status: Junior ================================================= ================================================= Name: Minnie Mouse Address: 127 Disney World, Orlando, FL 32801 Phone Number: (321)123-4571 Email: minniemouse@disney.com Status: Sophomore =================================================
Your program will be submitted to the Curator system. You will have five submissions. You will not be given more submissions for any reason! Your submitted program will be graded by the GTA. There will be no automatic grading for any project in this course. You will receive your grade via email from the class account cs1054@cs.vt.edu in the form of a grade-sheet. This project will be evaluated for style and design as well as correctness. Make sure you follow the standard Java conventions for indentation, variable names and documentation in your program. Look at programs 1 and 2 for examples of acceptable documentation. 60 of the 80 points will be correctness, while 20 points will be for style and documentation.
For this project, create a folder called P6. Put all the three required files Student.java, Person.java and Test.java in that folder. Zip or compress that folder. You can do this in Windows by right-clicking on the folder, then selecting "Send to" and finally, "Compressed (zipped) folder". You will submit this P6.zip file that gets created to the curator. As indicated earlier, you will submit this assignment to the Curator System (read the Student Guide). Instructions for submitting are contained in the Student Guide. You will be allowed up to five submissions for this assignment. Use them wisely. Test your program thoroughly before submitting it. It is advisable to show your program to the instructor or the TA to make sure it works correctly before submitting it. The Student Guide can be found at: http://www.cs.vt.edu/curator/
/**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Your Name * Your PID ****************************************************************************/Failure to include this pledge in a submission is a violation of the Honor Code.