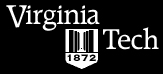
You will start using arrays in this project.
public static int[][] multiplyMatrix(int[][] a, int[][] b)To multiply matrix a by matrix b, the number of columns in a must be the same as the number of rows in b, and the two matrices must have elements of the same or compatible types. Let c be the result of the multiplication, and a, b, and c are denoted as follows:
3 3 1 2 3 4 5 6 7 8 9The first line indicates the dimensions of the matrix. If matrix1.txt was the above file and matrix2.txt looked like this:
3 3 1 0 0 0 1 0 0 0 1the result of the matrix multiplication should be stored in a file called matrixproduct.txt. For the above two input files, the output would look exactly the same as matrix1.txt, since the 2nd matrix is the identity matrix and would be
3 3 1 2 3 4 5 6 7 8 9You should print out the dimensions of the product matrix as the first line of the output file.
String matrixFilename1 = "matrix1.txt"; Scanner matrixScanner1 = new Scanner(new File(matrixFilename1));
String matrixProductFilename = "matrixProduct.txt"; PrintWriter matrixProduct = new PrintWriter(new File(matrixProductFilename));
Your program will be submitted to the Curator system. You will have five submissions. You will not be given more submissions for any reason! Your submitted program will be graded by the GTA. There will be no automatic grading for any project in this course. You will receive your grade via email from the class account cs1054@cs.vt.edu in the form of a grade-sheet. This project will be evaluated for style and design as well as correctness. Make sure you follow the standard Java conventions for indentation, variable names and documentation in your program. Look at programs 1 and 2 for examples of acceptable documentation. 60 of the 80 points will be correctness, while 20 points will be for style and documentation.
As indicated earlier, you will submit this assignment to the Curator System (read the Student Guide). Instructions for submitting are contained in the Student Guide. You will be allowed up to five submissions for this assignment. Use them wisely. Test your program thoroughly before submitting it. It is advisable to show your program to the instructor or the TA to make sure it works correctly before submitting it. Make sure that your program produces correct results for every sample input file posted on the course website. The Student Guide can be found at: http://www.cs.vt.edu/curator/
/**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Your Name * Your PID ****************************************************************************/Failure to include this pledge in a submission is a violation of the Honor Code.