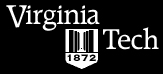
For the second programming project, you will be given most of the Java source code for a working program. The given source code includes all the necessary variable declarations and the mandatory input and output statements. Your task is to complete the given program by adding the Java statements needed to perform the specified calculations. You may modify the given program source code as much as you like. At minimum you must add the calculations. Just be careful if you make other changes since your output must match the formatting of the sample output exactly.
40 40 //Circle's center 40 // Circle's radius
Programmer: Mir Farooq Ali CS 1054 Summer I 2005 Project 2 Bounding Box Coordinates ------------------------ Top Right: (80,80) Top Left: (0,80) Bottom Left: (0,0) Bottom Right: (80,0) Areas and Perimeters -------------------- Circle Area: 5024.00 Circle Perimeter: 251.20 Bounding box Area: 6400.00 Bounding box Perimeter: 320.00Additional samples of input and correct output are available on the course website.
Your program will be submitted to the Curator system. You will have five submissions. You will not be given more submissions for any reason! Your submitted program will be graded by the GTA. There will not be any automatic grading for any project in this course. You will receive your grade via email from the class account cs1054@cs.vt.edu in the form of a grade-sheet. The grade for the project for future assignments include some points for style and design. This program will only be graded for correctness.
You will submit this assignment to the Curator System (read the Student Guide). Instructions for submitting are contained in the Student Guide. You will be allowed up to five submissions for this assignment. Use them wisely. Test your program thoroughly before submitting it. It is advisable to show your program to the instructor or the TA to make sure it works correctly before submitting it. Make sure that your program produces correct results for every sample input file posted on the course website. The Student Guide can be found at: http://www.cs.vt.edu/curator/
The comments used in this assignment are typical of what will be expected for all your projects. Program Compilation Your program must compile and run under JDK 1.5. Pledge Every program submission for this class must include an Honor Code pledge. Specifically, you must always include the following pledge statement in the header comment for your program:
/**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Your Name * Your PID ****************************************************************************/Failure to include this pledge in a submission is a violation of the Honor Code.
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; import java.util.Scanner; /**************************************************************************** * Project 2 for CS 1054 Summer 1 2005 * Circle calculations program * * File: Program2.java * Programmer: Mir Farooq Ali * Java Platform: JDK 1.5.0 * Compiler: Eclipse 3.1.0 * Last modified: June 1, 2005 * * Purpose: This program computes the coordinates of a bounding box of a circle * given its center and radius. It also calculates the area and perimeter * of the circle and the bounding box. The program then writes a summary of its * findings to an output file called analysis.txt * ****************************************************************************/ /**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Mir Farooq Ali * miali ****************************************************************************/ public class Program2 { public static void main(String[] args) throws FileNotFoundException { final double PI = 3.14; Scanner dataFile = new Scanner(new File("CircleData.txt")); PrintWriter logFile = new PrintWriter("Analysis.txt"); int xCenter, // X-coordinate of the circle's center yCenter, // Y-coordinate of the circle's center radius; // Radius of the circle int xTopRight, // X-coordinate of the top right corner of bounding box yTopRight, // Y-coordinate of the top right corner of bounding box xTopLeft, // X-coordinate of the top left corner of bounding box yTopLeft, // Y-coordinate of the top left corner of bounding box xBottomLeft, // X-coordinate of the bottom left corner of bounding box yBottomLeft, // Y-coordinate of the bottom left corner of bounding box xBottomRight,// X-coordinate of the bottom right corner of bounding box yBottomRight;// Y-coordinate of the bottom right corner of bounding box double circleArea, // Area of the circle circlePerimeter, // Perimeter of the circle bbArea, // Bounding Box area bbPerimeter; // Bounding Box perimeter // Write an identification header to the output file: logFile.print("Programmer: "); logFile.println("Mir Farooq Ali"); // Change this to YOUR name. logFile.println("CS 1054 Summer I 2005 Project 2"); logFile.println(); // Read the coordinates of the circle's center: xCenter = dataFile.nextInt(); yCenter = dataFile.nextInt(); dataFile.useDelimiter("\\n"); String temp = dataFile.next(); dataFile.useDelimiter("\\s"); radius = dataFile.nextInt(); // Check for an invalid circle if (radius < 0) { logFile.println("The given radius for the circle is invalid."); System.exit(0); } // You must add the following calculations: // Calculate the coordinates of the bounding box // Calculate the circle's area // Calculate the circle's perimeter // Calculate the bounding box's area // Calculate the bounding box's perimeter // Write out the analysis: logFile.println("Bounding Box Coordinates"); logFile.println("------------------------"); logFile.println("Top Right: (" + xTopRight + ',' + yTopRight + ')'); logFile.println("Top Left: (" + xTopLeft + ',' + yTopLeft + ')'); // xTopLeft changed to yTopLeft logFile.println("Bottom Left: (" + xBottomLeft + ',' + yBottomLeft + ')'); logFile.println("Bottom Right: (" + xBottomRight + ',' + yBottomRight + ')'); logFile.println(); logFile.println("Areas and Perimeters"); logFile.println("--------------------"); logFile.printf("Circle Area: %6.2f \n", circleArea); logFile.printf("Circle Perimeter: %6.2f \n", circlePerimeter); logFile.printf("Bounding box Area: %6.2f \n", bbArea); logFile.printf("Bounding box Perimeter: %6.2f \n", bbPerimeter); dataFile.close(); logFile.close(); } }