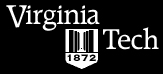
Hopefully, by dealing with these issues now, they should be less of a shock when you have to actually author your first program in Project 2. For now, do not worry about trying to figure out how the program works. Some features of this program will be covered early in the semester, some very late in the semester, and some not at all. Do not expect to understand everything.
This program converts temperature scales. It must be named "Program1.java". It accepts input from a file, called "celsius_in.txt", which holds five temperatures in the Celsius scale. It then converts the temperatures to Celsius and writes appropriate output to another file, called "fahrenheit_out.txt". There are a few points in the program where you must substitute your personal information for what is written in the code below. Those places in the code are indicated in red color. For example, you should replace occurrences of my name (Mir Farooq Ali) with your own name. Similarly, replace the dates (also indicated in red color) in the code with the dates when you work on your project, etc. Test files will be posted on the website so that you can test your program’s correctness. I also suggest that you create your own test input files and check your program with them. The posted test files are not meant to be a guarantee of a completely correct program.
Your program will be submitted to the Curator system. You will have five submissions. You will not be given more submissions for any reason! Your submitted program will be graded by the GTA. There will not be any automatic grading for any project in this course. You will receive your grade via email from the class account cs1054@cs.vt.edu in the form of a grade-sheet. The grade for the project for future assignments include some points for style and design. This program will only be graded for correctness.
You will submit this assignment to the Curator System (read the Student Guide). Instructions for submitting are contained in the Student Guide. You will be allowed up to five submissions for this assignment. Use them wisely. Test your program thoroughly before submitting it. It is advisable to show your program to the instructor or the TA to make sure it works correctly before submitting it. Make sure that your program produces correct results for every sample input file posted on the course website. The Student Guide can be found at: http://www.cs.vt.edu/curator/
The comments used in this assignment are typical of what will be expected for all your projects. Program Compilation Your program must compile and run under JDK 1.5. Pledge Every program submission for this class must include an Honor Code pledge. Specifically, you must always include the following pledge statement in the header comment for your program:
/**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Your Name * Your PID ****************************************************************************/Failure to include this pledge in a submission is a violation of the Honor Code.
import java.io.*; import java.util.Scanner; /**************************************************************************** * Project 1 for CS 1054 Summer 1 2005 * Temperature Converter Program * * File: Program1.java * Programmer: Mir Farooq Ali * Java Platform: JDK 1.5.0 * Compiler: Eclipse 3.1.0 * Last modified: May 25, 2005 * * Purpose: This program takes input from a file named "celsius_in.txt", * which contains five lines, each containing a single decimal * value representing a temperature in the Celsius scale. * This program converts those five temperatures to Fahrenheit * scale, and sends results to a file called "fahrenheit_out.txt". ****************************************************************************/ /**************************************************************************** * * On my honor: * * - I have not discussed the Java language code in my program with * anyone other than my instructor or the teaching assistants * assigned to this course. * * - I have not used Java language code obtained from another student, * or any other unauthorized source, either modified or unmodified. * * - If any Java language code or documentation used in my program * was obtained from another source, such as a text book or course * notes, that has been clearly noted with a proper citation in * the comments of my program. * * - I have not designed this program in such a way as to defeat or * interfere with the normal operation of the Curator System. * * Mir Farooq Ali * miali ****************************************************************************/ public class Program1 { /************************************************************************* * Project 1 for CS 1054 Summer 1 - 2005 * Temperature Converter Program * * Programmer: Mir Farooq Ali * Java Platform: JDK 1.5.0 * IDE: Eclipse 3.1.0 * Last modified: May 25, 2005 * * Purpose: This program takes input from a file named "celsius_in.txt", * which contains five lines, each containing a single decimal * value representing a temperature in the Celsius scale. * This program converts those five temperatures to Fahrenheit * scale, and sends results to a file called "fahrenheit_out.txt". * Method: main * Programmer Mir Farooq Ali * Arguments: String array; ignored * Returns: void * Throws: FileNotFound & IO Exceptions * Last Modified: May 25, 2005 * * Purpose: This method declares variables, reads and decodes * input, and generates and writes appropriate output. ***********************************************************************/ public static void main(String args[]) throws FileNotFoundException, IOException { // String objects holding the input and output filenames String myInFileName = "celsius_in.txt"; String myOutFileName = "fahrenheit_out.txt"; // five decimal Celsius temperatures, as determined from input double cTemp1, cTemp2, cTemp3, cTemp4, cTemp5; //********************************************************************** //* SET UP THE INPUT SCANNER * //********************************************************************** Scanner inputScanner = new Scanner(new File(myInFileName)); //********************************************************************** //********************************************************************** //* SET UP THE OUTPUT PRINT WRITER * //********************************************************************** PrintWriter outputWriter = new PrintWriter(new File(myOutFileName)); //********************************************************************** // Read the input values from the input file cTemp1 = inputScanner.nextDouble(); cTemp2 = inputScanner.nextDouble(); cTemp3 = inputScanner.nextDouble(); cTemp4 = inputScanner.nextDouble(); cTemp5 = inputScanner.nextDouble(); // write the headers out outputWriter.println("Programmer: Mir Farooq Ali"); outputWriter.println("CS1054: Project 1 Summer 2005"); outputWriter.println(); // write the messages out outputWriter.printf(" %6.2f Celsius = %6.2f Fahrenheit\n", cTemp1, celsToFahrs(cTemp1)); outputWriter.printf(" %6.2f Celsius = %6.2f Fahrenheit\n", cTemp2, celsToFahrs(cTemp2)); outputWriter.printf(" %6.2f Celsius = %6.2f Fahrenheit\n", cTemp3, celsToFahrs(cTemp3)); outputWriter.printf(" %6.2f Celsius = %6.2f Fahrenheit\n", cTemp4, celsToFahrs(cTemp4)); outputWriter.printf(" %6.2f Celsius = %6.2f Fahrenheit\n", cTemp5, celsToFahrs(cTemp5)); // close the input scanner and output writer inputScanner.close(); outputWriter.close(); } /************************************************************* * Method: celsToFahrs * Programmer Mir Farooq Ali * Arguments: a Celsius temperature as a double * Returns: the corresponding Celsius temperature as a double * Last Modified: May 25, 2005 * * Purpose: This method does arithmetic temperature * conversion from Celsius to Fahrenheit scales. **************************************************************/ static double celsToFahrs(double cels){ return (cels*9.0)/5.0 + 32; } }