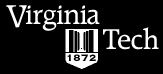
This exercise is to introduce you to Java applets. Applets are Java programs that run within a web browser and allow us to add “dynamic” content to our websites. We will start with a simple applet and practice using some of the graphical classes.
import java.awt.*; import java.applet.*; public class FirstApplet extends Applet { public void paint(Graphics g) { Color c; c = new Color(180,10,120); g.setColor(c); g.fillOval(20,20,60,30); } }
g.drawOval(x, y, ovalWidth –1, ovalHeight – 1);or, this statement that paints a filled oval of the same size
g.fillOval(x, y, ovalWidth, ovalHeight);(The reason for subtracting one for the drawOval method is that the painting system draws lines just below the specified dimensions, instead of within the specified dimensions. The same rule of specifying one less than the desired width applies to other drawXxx methods. For the fillXxx methods, on the other hand, you specify exactly the desired width and height in pixels.) The drawLine method also takes four arguments with the arguments being four integers specifying x, y coordinates of the start and end of the line:
g.drawLine(xstart, ystart, xend, yend);The program above draws a filled oval with an x position of 20, a y position of 20, a width of 60 and a height of 30. [code snippet: g.fillOval(20,20,60,30); ] Also in the program, we specify a color by defining a new Color c and defining it to be the color formed by mixing 20 units of red, 120 units of green and 160 units of blue (on a scale of 0 to 255). This produced a dark pink color. By varying the amounts of red, blue, and green you can define your own color. However, if you just want to use standard colors, there is a simpler way of doing this:
g.setColor(Color.yellow);The argument to setColor using preset colors can be any of the following:
Color.black Color.blue Color.cyan Color.darkGray Color.gray Color.green Color.lightGray Color.magenta Color.orange Color.pink Color.red Color.white Color.yellow